A flowchart is a diagram that illustrates the computational steps used by one method. That one method may be the main method or another method in a program, but one flowchart is for just one method. Methods start and stop. The main method starts automatically when the program starts running, and its end means the program is done. Other methods start by being called and end by either returning a value (value methods) or simply having no store statements to execute (void methods). In a flowchart, we put Start and End in ovals, and then use other symbols in between to illustrate each statement in turn. The “flow” is shown by connecting symbols with arrows.
Here are the symbols:
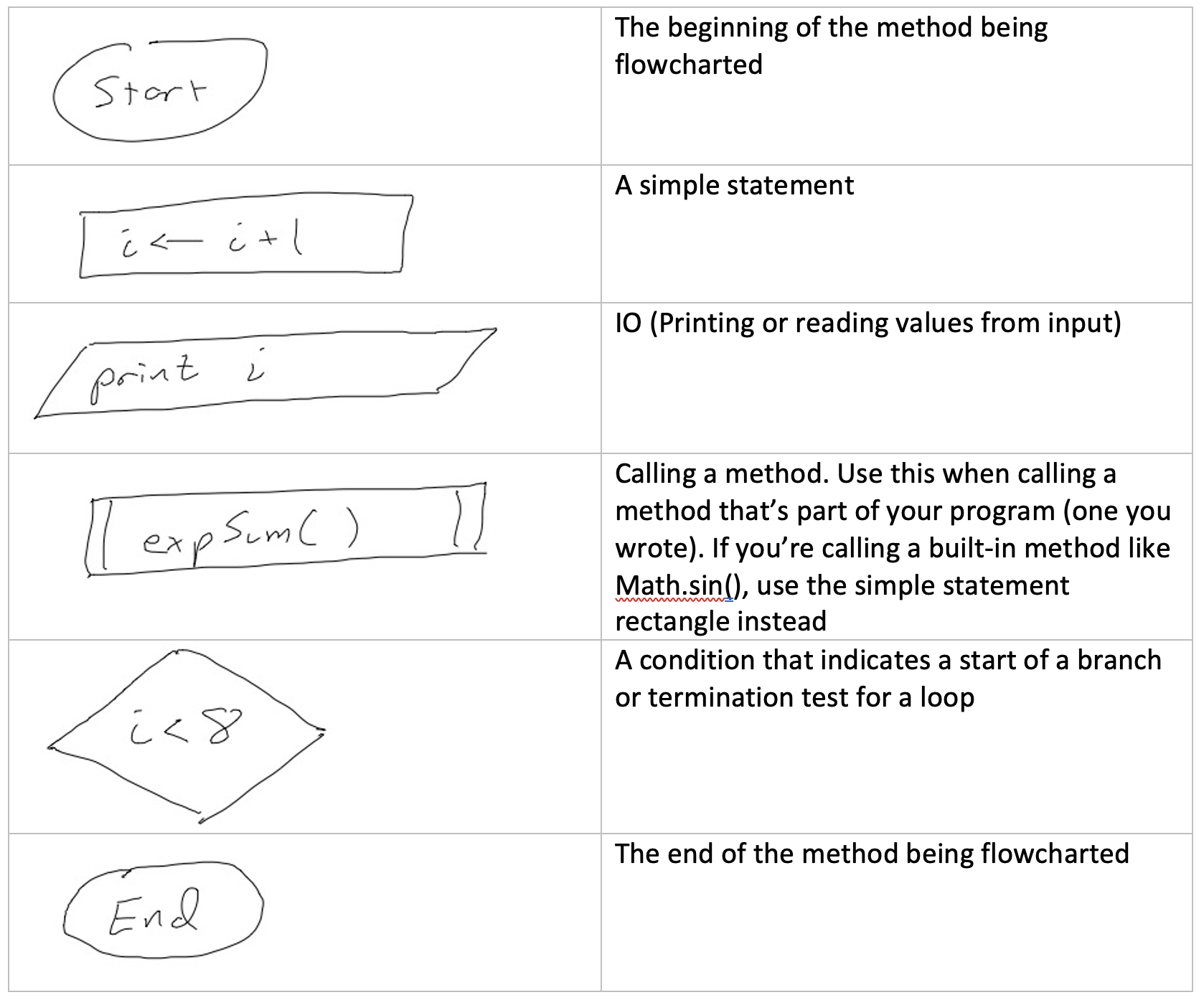
One thing to note is that flowcharts are not Java-specific. They’re much older than Java, and they’re not really for any particular programming language. They’re for all them. People designing challenging programs may flowchart first before getting into the more detailed process of coding. Because of this independence from Java, many flowcharters will include statements like i ← i + 1 instead i = i +1. Similarly, English words like print rather than Java-specific names like System.out.print are often used.
Simple flow, one statement after another
If your method has no branches or loops, then it simply executes one statement after another. The flowchart will have Start at the top, with the first statement beneath that, the second statement underneath the first, and so on until End at the bottom. Each statement will flow into the next with a downward pointing arrow.
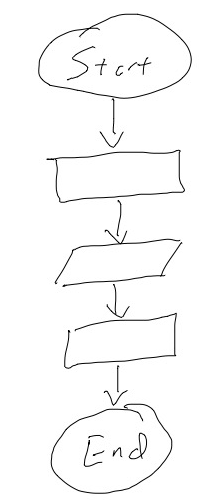
Branches
A branch uses a test to decide which way the flow will go. The test is something that is true or false (either a comparison of two values like 2 < 3 or something more complicated like x < y || y > z). If the test is true, the flow goes one direction, and if the test is false, the flow goes another. So branches have two outgoing arrows instead of the one that the other symbols get.
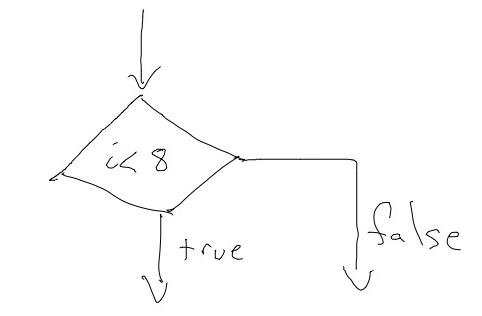
We’ve seen four Java concepts that use branches: if statements, for loops, while loops, and do loops. They all use the diamond symbol in the flowchart because they all have tests for a branch. The loops have a termination testto determine if the iteration keeps going, and if statements use the test to decide whether or not to follow the true or the false branch.
If statements
In Java, the else (false branch) is optional. An if/else in our method looks like the flow splitting into two sets of statements (one for the if and one for the else), and then the flow joins together again after both branches’ statements.
Consider the Java code:
if (i < 8) { i = i + 2; } else { i = i -2; } // rest of the method
and its corresponding flowchart below. This example has only 1 statement in each branch, but remember each branch can have many of them. You just keep stacking one statement on top of another and connecting with arrows until the branch is done.
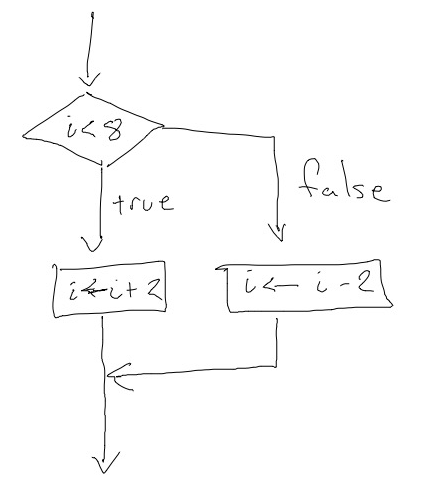
If there is no else branch, then in the flowchart there will be no statements for that branch. The false branch will just be an arrow that goes around the true branches’ statements and then joins back up to the main flow.
Consider this Java code:
if (i < 8) { i = i + 2; } // rest of the method
and its corresponding flowchart below. There’s no else, so when the true branch doesn’t happen, the flow continues to the rest of the code. Our diamond for the branch still has two outbound arrows, but the false one doesn’t have any statements because there’s no else statements to diagram!
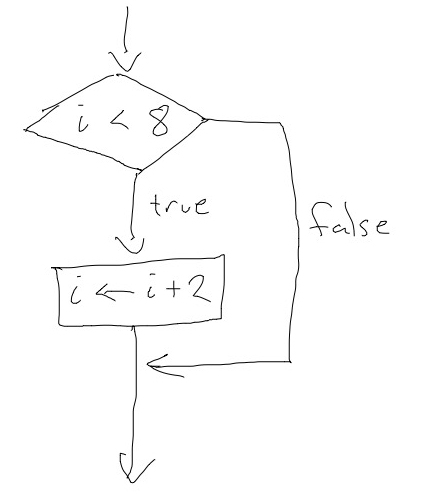
Loops
Loops differ from if statements in that they can happen more than once. They loop back. So when a loop is shown in a flowchart, the flow will loop back around. For and while loops have their termination tests before the body of the loop and do loops have their test at the end. As far as flowcharts are concerned for and while loops are identical! Note that the words for, while, or do don’t show up in the flowchart. The loop is the test in a diamond, the branching of the flow, and the looping back to allow iteration.
Here are two different snippets of Java code, one with a for loop and one with a while.
for (i = 0; i < 8; i++) System.out.println(i); // rest of the method
and
i = 1; while (i < 8) { System.out.println(i); i = i+1; } // rest of the method
and their corresponding flowchart below:
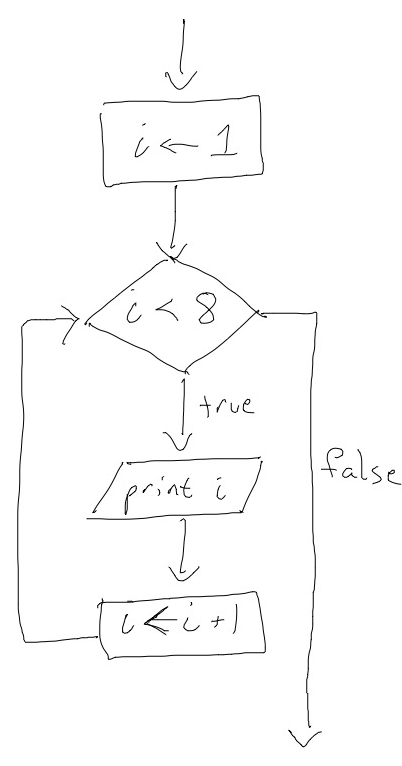
With for and while loops, we can’t tell which is which from the flowchart! We’ve seen that we can code a loop in either, but that the for loop is easiest or best when we have some idea of the number of iterations because the for loop syntax automates the loop counter variable for us.
The last one to consider is a do-while loop where the termination test is at the end. After all this info above, it’s mainly about just following the rules of the symbols. Here’s some Java code
i = 1; do { System.out.println(i); i = i + 1; while (i < 8); // rest of the method
With this one, we just put the diamond last, and keep track to remember that when the test is true, it loops back, and when the test is false, it flows on to the rest of the method
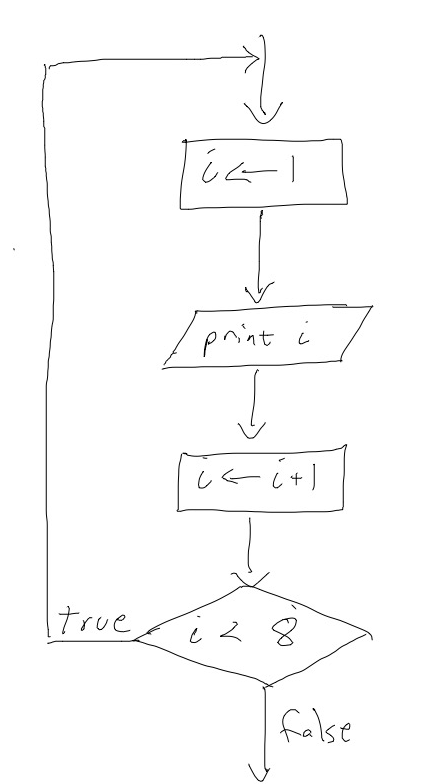